diff --git a/src/routes/api/v1/services/deploy/code-server/index.ts b/src/routes/api/v1/services/deploy/code-server/index.ts
new file mode 100644
index 000000000..81ab88e67
--- /dev/null
+++ b/src/routes/api/v1/services/deploy/code-server/index.ts
@@ -0,0 +1,74 @@
+import type { Request } from '@sveltejs/kit';
+import yaml from 'js-yaml';
+import { promises as fs } from 'fs';
+import { docker } from '$lib/api/docker';
+import { baseServiceConfiguration } from '$lib/api/applications/common';
+import { cleanupTmp, execShellAsync } from '$lib/api/common';
+
+export async function post(request: Request) {
+ let { baseURL } = request.body;
+ const traefikURL = baseURL;
+ baseURL = `https://${baseURL}`;
+ const workdir = '/tmp/code-server';
+ const deployId = 'code-server';
+ // const environment = [
+ // { name: 'DOCKER_USER', value: 'root' }
+
+ // ];
+ // const generateEnvsCodeServer = {};
+ // for (const env of environment) generateEnvsCodeServer[env.name] = env.value;
+
+ const stack = {
+ version: '3.8',
+ services: {
+ [deployId]: {
+ image: 'codercom/code-server',
+ command: 'code-server --disable-telemetry',
+ networks: [`${docker.network}`],
+ volumes: [`${deployId}-code-server-data:/home/coder`],
+ // environment: generateEnvsCodeServer,
+ deploy: {
+ ...baseServiceConfiguration,
+ labels: [
+ 'managedBy=coolify',
+ 'type=service',
+ 'serviceName=code-server',
+ 'configuration=' +
+ JSON.stringify({
+ baseURL
+ }),
+ 'traefik.enable=true',
+ 'traefik.http.services.' + deployId + '.loadbalancer.server.port=8080',
+ 'traefik.http.routers.' + deployId + '.entrypoints=websecure',
+ 'traefik.http.routers.' +
+ deployId +
+ '.rule=Host(`' +
+ traefikURL +
+ '`) && PathPrefix(`/`)',
+ 'traefik.http.routers.' + deployId + '.tls.certresolver=letsencrypt',
+ 'traefik.http.routers.' + deployId + '.middlewares=global-compress'
+ ]
+ }
+ }
+ },
+ networks: {
+ [`${docker.network}`]: {
+ external: true
+ }
+ },
+ volumes: {
+ [`${deployId}-code-server-data`]: {
+ external: true
+ },
+ },
+ };
+ await execShellAsync(`mkdir -p ${workdir}`);
+ await fs.writeFile(`${workdir}/stack.yml`, yaml.dump(stack));
+ await execShellAsync('docker stack rm code-server');
+ await execShellAsync(`cat ${workdir}/stack.yml | docker stack deploy --prune -c - ${deployId}`);
+ cleanupTmp(workdir);
+ return {
+ status: 200,
+ body: { message: 'OK' }
+ };
+}
diff --git a/src/routes/api/v1/services/deploy/code-server/password.ts b/src/routes/api/v1/services/deploy/code-server/password.ts
new file mode 100644
index 000000000..825dcca14
--- /dev/null
+++ b/src/routes/api/v1/services/deploy/code-server/password.ts
@@ -0,0 +1,25 @@
+import { execShellAsync } from '$lib/api/common';
+import type { Request } from '@sveltejs/kit';
+import yaml from "js-yaml"
+
+export async function get(request: Request) {
+ // const { POSTGRESQL_USERNAME, POSTGRESQL_PASSWORD, POSTGRESQL_DATABASE } = JSON.parse(
+ // JSON.parse(
+ // await execShellAsync(
+ // "docker service inspect code-server_code-server --format='{{json .Spec.Labels.configuration}}'"
+ // )
+ // )
+ // ).generateEnvsPostgres;
+ const containers = (await execShellAsync("docker ps -a --format='{{json .Names}}'"))
+ .replace(/"/g, '')
+ .trim()
+ .split('\n');
+ const codeServer = containers.find((container) => container.startsWith('code-server'));
+ const configYaml = yaml.load(await execShellAsync(
+ `docker exec ${codeServer} cat /home/coder/.config/code-server/config.yaml`
+ ))
+ return {
+ status: 200,
+ body: { message: 'OK', password: configYaml.password }
+ };
+}
diff --git a/src/routes/api/v1/services/deploy/minio/index.ts b/src/routes/api/v1/services/deploy/minio/index.ts
new file mode 100644
index 000000000..c4a247e0a
--- /dev/null
+++ b/src/routes/api/v1/services/deploy/minio/index.ts
@@ -0,0 +1,77 @@
+import type { Request } from '@sveltejs/kit';
+import yaml from 'js-yaml';
+import generator from 'generate-password';
+import { promises as fs } from 'fs';
+import { docker } from '$lib/api/docker';
+import { baseServiceConfiguration } from '$lib/api/applications/common';
+import { cleanupTmp, execShellAsync } from '$lib/api/common';
+
+export async function post(request: Request) {
+ let { baseURL } = request.body;
+ const traefikURL = baseURL;
+ baseURL = `https://${baseURL}`;
+ const workdir = '/tmp/minio';
+ const deployId = 'minio';
+ const secrets = [
+ { name: 'MINIO_ROOT_USER', value: generator.generate({ length: 12, numbers: true, strict: true }) },
+ { name: 'MINIO_ROOT_PASSWORD', value: generator.generate({ length: 24, numbers: true, strict: true }) }
+
+ ];
+ const generateEnvsMinIO = {};
+ for (const secret of secrets) generateEnvsMinIO[secret.name] = secret.value;
+
+ const stack = {
+ version: '3.8',
+ services: {
+ [deployId]: {
+ image: 'minio/minio',
+ command: 'server /data',
+ networks: [`${docker.network}`],
+ environment: generateEnvsMinIO,
+ volumes: [`${deployId}-minio-data:/data`],
+ deploy: {
+ ...baseServiceConfiguration,
+ labels: [
+ 'managedBy=coolify',
+ 'type=service',
+ 'serviceName=minio',
+ 'configuration=' +
+ JSON.stringify({
+ baseURL,
+ generateEnvsMinIO
+ }),
+ 'traefik.enable=true',
+ 'traefik.http.services.' + deployId + '.loadbalancer.server.port=9000',
+ 'traefik.http.routers.' + deployId + '.entrypoints=websecure',
+ 'traefik.http.routers.' +
+ deployId +
+ '.rule=Host(`' +
+ traefikURL +
+ '`) && PathPrefix(`/`)',
+ 'traefik.http.routers.' + deployId + '.tls.certresolver=letsencrypt',
+ 'traefik.http.routers.' + deployId + '.middlewares=global-compress'
+ ]
+ }
+ }
+ },
+ networks: {
+ [`${docker.network}`]: {
+ external: true
+ }
+ },
+ volumes: {
+ [`${deployId}-minio-data`]: {
+ external: true
+ },
+ },
+ };
+ await execShellAsync(`mkdir -p ${workdir}`);
+ await fs.writeFile(`${workdir}/stack.yml`, yaml.dump(stack));
+ await execShellAsync('docker stack rm minio');
+ await execShellAsync(`cat ${workdir}/stack.yml | docker stack deploy --prune -c - ${deployId}`);
+ cleanupTmp(workdir);
+ return {
+ status: 200,
+ body: { message: 'OK' }
+ };
+}
diff --git a/src/routes/dashboard/applications.svelte b/src/routes/dashboard/applications.svelte
index d82265126..3139a0cea 100644
--- a/src/routes/dashboard/applications.svelte
+++ b/src/routes/dashboard/applications.svelte
@@ -37,7 +37,7 @@
{#each $dashboard.applications.deployed as application}
{
goto(
`/application/${application.configuration.repository.organization}/${application.configuration.repository.name}/${application.configuration.repository.branch}/configuration`
diff --git a/src/routes/dashboard/databases.svelte b/src/routes/dashboard/databases.svelte
index 44c772bb9..c8d3cad80 100644
--- a/src/routes/dashboard/databases.svelte
+++ b/src/routes/dashboard/databases.svelte
@@ -45,7 +45,7 @@
goto(`/database/${database.configuration.general.deployId}/configuration`)}
>
{#if database.configuration.general.type == 'mongodb'}
diff --git a/src/routes/dashboard/services.svelte b/src/routes/dashboard/services.svelte
index ca4fd1b5f..9331b1aa8 100644
--- a/src/routes/dashboard/services.svelte
+++ b/src/routes/dashboard/services.svelte
@@ -37,7 +37,7 @@
on:click={() => goto(`/service/${service.serviceName}/configuration`)}
>
{#if service.serviceName == 'plausible'}
@@ -58,6 +58,24 @@
/>
NocoDB
+ {:else if service.serviceName == 'code-server'}
+
+
+
+
VSCode Server
+
+ {:else if service.serviceName == 'minio'}
+
+
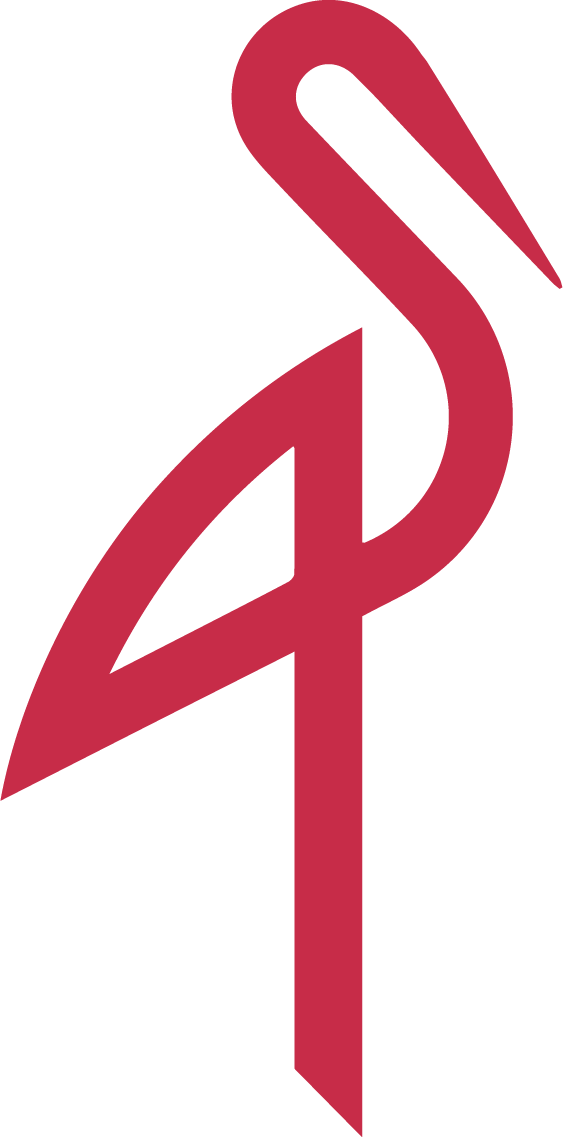
+
+
MinIO
+
{/if}
diff --git a/src/routes/service/[name]/configuration.svelte b/src/routes/service/[name]/configuration.svelte
index 1162a9ec5..e2ea07399 100644
--- a/src/routes/service/[name]/configuration.svelte
+++ b/src/routes/service/[name]/configuration.svelte
@@ -8,6 +8,8 @@
import Loading from '$components/Loading.svelte';
import Plausible from '$components/Service/Plausible.svelte';
import { browser } from '$app/env';
+ import CodeServer from '$components/Service/CodeServer.svelte';
+ import MinIo from '$components/Service/MinIO.svelte';
let service = {};
async function loadServiceConfig() {
if ($page.params.name) {
@@ -33,6 +35,10 @@
Plausible Analytics
{:else if $page.params.name === 'nocodb'}
NocoDB
+ {:else if $page.params.name === 'code-server'}
+
VSCode Server
+ {:else if $page.params.name === 'minio'}
+
MinIO
{/if}
@@ -48,6 +54,28 @@
class="w-8 mx-auto"
src="https://cdn.coollabs.io/assets/coolify/services/nocodb/nocodb.png"
/>
+ {:else if $page.params.name === 'code-server'}
+
+ {:else if $page.params.name === 'minio'}
+
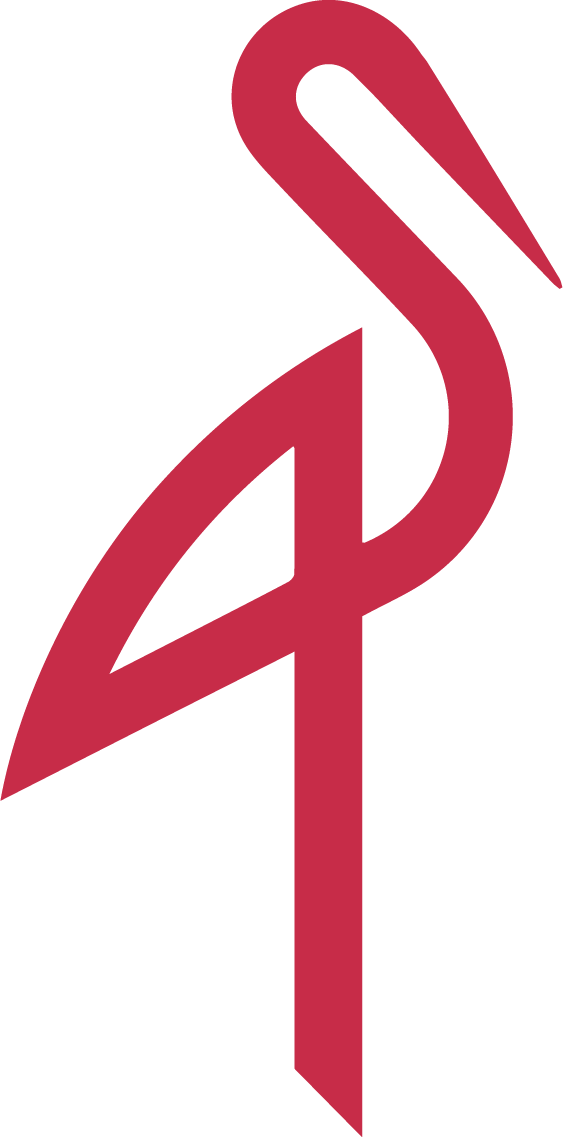
{/if}
@@ -74,6 +102,10 @@
{:else if $page.params.name === 'nocodb'}
Nothing to show here. Enjoy using NocoDB!
+ {:else if $page.params.name === 'code-server'}
+
+ {:else if $page.params.name === 'minio'}
+
{/if}
diff --git a/src/routes/service/new/[type]/__layout.svelte b/src/routes/service/new/[type]/__layout.svelte
index d3007cbf4..1846ab576 100644
--- a/src/routes/service/new/[type]/__layout.svelte
+++ b/src/routes/service/new/[type]/__layout.svelte
@@ -1,8 +1,6 @@
@@ -13,7 +14,7 @@
{#if $page.path === '/service/new'}
goto('/service/new/plausible')}
>
![]()
Plausible Analytics
goto('/service/new/nocodb')}
>
NocoDB
+
goto('/service/new/code-server')}
+ >
+
+
+
VSCode Server
+
+
goto('/service/new/minio')}
+ >
+
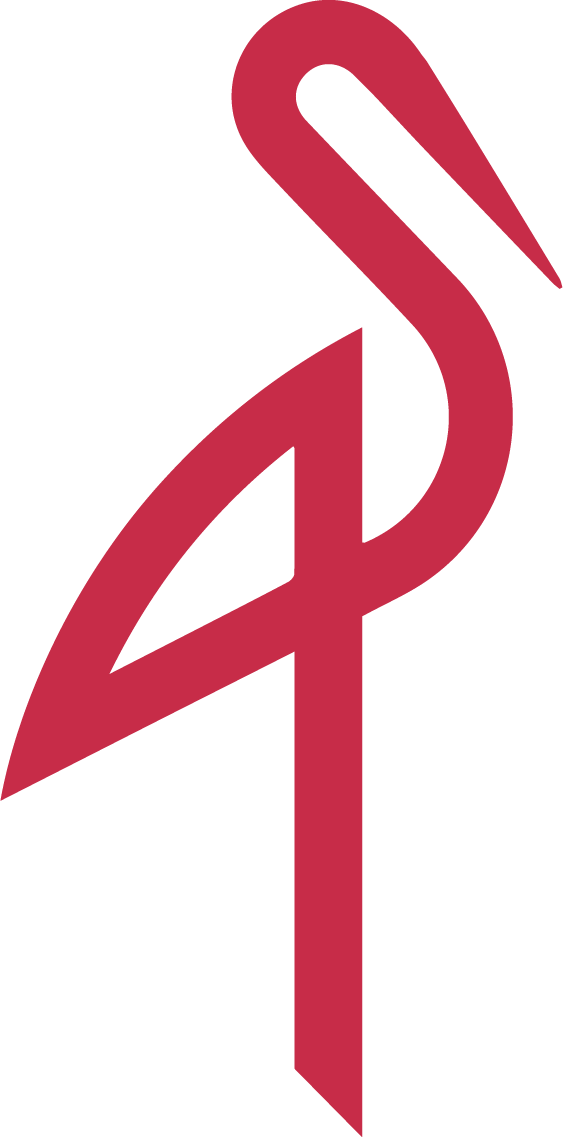
+
+
MinIO
+
{/if}
diff --git a/src/routes/settings.svelte b/src/routes/settings.svelte
index d6cc73d3d..b198bb8ac 100644
--- a/src/routes/settings.svelte
+++ b/src/routes/settings.svelte
@@ -83,7 +83,7 @@
>
Use setting
@@ -143,7 +143,7 @@
>
Use setting